-
- All Implemented Interfaces:
- Paint, Transparency
public class
GradientPaint
extends java.lang.Object
implements
Paint
The
GradientPaint
class provides a way to fill
a
Shape
with a linear color gradient pattern.
If
Point
P1 with
Color
C1 and
Point
P2 with
Color
C2 are specified in user space, the
Color
on the P1, P2 connecting line is proportionally
changed from C1 to C2. Any point P not on the extended P1, P2
connecting line has the color of the point P' that is the perpendicular
projection of P on the extended P1, P2 connecting line.
Points on the extended line outside of the P1, P2 segment can be colored
in one of two ways.
-
If the gradient is cyclic then the points on the extended P1, P2
connecting line cycle back and forth between the colors C1 and C2.
-
If the gradient is acyclic then points on the P1 side of the segment
have the constant
Color
C1 while points on the P2 side
have the constant Color
C2.
- See Also:
Paint
,
Graphics2D.setPaint(java.awt.Paint)
-
-
|
Modifier and Type |
Field and Description |
 |
BITMASK   |
static int BITMASK
Represents image data that is guaranteed to be either completely
opaque, with an alpha value of 1.0, or completely transparent,
with an alpha value of 0.0.
public static final int BITMASK
-
Represents image data that is guaranteed to be either completely
opaque, with an alpha value of 1.0, or completely transparent,
with an alpha value of 0.0.
- See Also:
- Constant Field Values
|
 |
OPAQUE   |
static int OPAQUE
Represents image data that is guaranteed to be completely opaque,
meaning that all pixels have an alpha value of 1.0.
public static final int OPAQUE
-
Represents image data that is guaranteed to be completely opaque,
meaning that all pixels have an alpha value of 1.0.
- See Also:
- Constant Field Values
|
 |
TRANSLUCENT   |
static int TRANSLUCENT
Represents image data that contains or might contain arbitrary
alpha values between and including 0.0 and 1.0.
public static final int TRANSLUCENT
-
Represents image data that contains or might contain arbitrary
alpha values between and including 0.0 and 1.0.
- See Also:
- Constant Field Values
|
-
|
Constructor and Description |
 |
GradientPaint   |
GradientPaint(float x1,
float y1,
Color color1,
float x2,
float y2,
Color color2)
Constructs a simple acyclic GradientPaint object.
public GradientPaint(float x1,
float y1,
Color color1,
float x2,
float y2,
Color color2)
-
Constructs a simple acyclic GradientPaint object.
- Parameters:
x1 - x coordinate of the first specified
Point in user spacey1 - y coordinate of the first specified
Point in user spacecolor1 - Color at the first specified
Point x2 - x coordinate of the second specified
Point in user spacey2 - y coordinate of the second specified
Point in user spacecolor2 - Color at the second specified
Point
- Throws:
java.lang.NullPointerException - if either one of colors is null
|
 |
GradientPaint   |
GradientPaint(float x1,
float y1,
Color color1,
float x2,
float y2,
Color color2,
boolean cyclic)
Constructs either a cyclic or acyclic GradientPaint
object depending on the boolean parameter.
public GradientPaint(float x1,
float y1,
Color color1,
float x2,
float y2,
Color color2,
boolean cyclic)
-
Constructs either a cyclic or acyclic GradientPaint
object depending on the boolean parameter.
- Parameters:
x1 - x coordinate of the first specified
Point in user spacey1 - y coordinate of the first specified
Point in user spacecolor1 - Color at the first specified
Point x2 - x coordinate of the second specified
Point in user spacey2 - y coordinate of the second specified
Point in user spacecolor2 - Color at the second specified
Point cyclic - true if the gradient pattern should cycle
repeatedly between the two colors; false otherwise
|
 |
GradientPaint   |
GradientPaint(java.awt.geom.Point2D pt1,
Color color1,
java.awt.geom.Point2D pt2,
Color color2)
Constructs a simple acyclic GradientPaint object.
public GradientPaint(java.awt.geom.Point2D pt1,
Color color1,
java.awt.geom.Point2D pt2,
Color color2)
-
Constructs a simple acyclic GradientPaint object.
- Parameters:
pt1 - the first specified Point in user spacecolor1 - Color at the first specified
Point pt2 - the second specified Point in user spacecolor2 - Color at the second specified
Point
- Throws:
java.lang.NullPointerException - if either one of colors or points
is null
|
 |
GradientPaint   |
GradientPaint(java.awt.geom.Point2D pt1,
Color color1,
java.awt.geom.Point2D pt2,
Color color2,
boolean cyclic)
Constructs either a cyclic or acyclic GradientPaint
object depending on the boolean parameter.
@ConstructorProperties(value={"point1","color1","point2","color2","cyclic"})
public GradientPaint(java.awt.geom.Point2D pt1,
Color color1,
java.awt.geom.Point2D pt2,
Color color2,
boolean cyclic)
-
Constructs either a cyclic or acyclic GradientPaint
object depending on the boolean parameter.
- Parameters:
pt1 - the first specified Point
in user spacecolor1 - Color at the first specified
Point pt2 - the second specified Point
in user spacecolor2 - Color at the second specified
Point cyclic - true if the gradient pattern should cycle
repeatedly between the two colors; false otherwise
- Throws:
java.lang.NullPointerException - if either one of colors or points
is null
|
-
|
Modifier and Type |
Method and Description |
 |
createContext   |
PaintContext createContext(java.awt.image.ColorModel cm,
Rectangle deviceBounds,
java.awt.geom.Rectangle2D userBounds,
java.awt.geom.AffineTransform xform,
RenderingHints hints)
Creates and returns a PaintContext used to
generate a linear color gradient pattern.
public PaintContext createContext(java.awt.image.ColorModel cm,
Rectangle deviceBounds,
java.awt.geom.Rectangle2D userBounds,
java.awt.geom.AffineTransform xform,
RenderingHints hints)
-
Creates and returns a PaintContext used to
generate a linear color gradient pattern.
See the specification of the
method in the Paint interface for information
on null parameter handling.
- Specified by:
createContext in interface Paint
- Parameters:
cm - the preferred ColorModel which represents the most convenient
format for the caller to receive the pixel data, or null
if there is no preference.deviceBounds - the device space bounding box
of the graphics primitive being rendered.userBounds - the user space bounding box
of the graphics primitive being rendered.xform - the AffineTransform from user
space into device space.hints - the set of hints that the context object can use to
choose between rendering alternatives.
- Returns:
- the
PaintContext for
generating color patterns. - See Also:
Paint ,
PaintContext ,
ColorModel ,
Rectangle ,
Rectangle2D ,
AffineTransform ,
RenderingHints
|
 |
getColor1   |
Returns the color C1 anchored by the point P1.
-
Returns the color C1 anchored by the point P1.
- Returns:
- a
Color object that is the color
anchored by P1.
|
 |
getColor2   |
Returns the color C2 anchored by the point P2.
-
Returns the color C2 anchored by the point P2.
- Returns:
- a
Color object that is the color
anchored by P2.
|
 |
getPoint1   |
java.awt.geom.Point2D getPoint1()
Returns a copy of the point P1 that anchors the first color.
public java.awt.geom.Point2D getPoint1()
-
Returns a copy of the point P1 that anchors the first color.
- Returns:
- a
Point2D object that is a copy of the point
that anchors the first color of this
GradientPaint .
|
 |
getPoint2   |
java.awt.geom.Point2D getPoint2()
Returns a copy of the point P2 which anchors the second color.
public java.awt.geom.Point2D getPoint2()
-
Returns a copy of the point P2 which anchors the second color.
- Returns:
- a
Point2D object that is a copy of the point
that anchors the second color of this
GradientPaint .
|
 |
getTransparency   |
int getTransparency()
Returns the transparency mode for this GradientPaint .
public int getTransparency()
-
Returns the transparency mode for this GradientPaint .
- Specified by:
getTransparency in interface Transparency
- Returns:
- an integer value representing this
GradientPaint
object's transparency mode. - See Also:
Transparency
|
 |
isCyclic   |
boolean isCyclic()
Returns true if the gradient cycles repeatedly
between the two colors C1 and C2.
public boolean isCyclic()
-
Returns true if the gradient cycles repeatedly
between the two colors C1 and C2.
- Returns:
true if the gradient cycles repeatedly
between the two colors; false otherwise.
|
-
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
This document was created by
Dulcet from the OpenJDK sources.
Copyright © 1993, 2012 Oracle and/or its affiliates. All rights reserved.
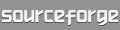